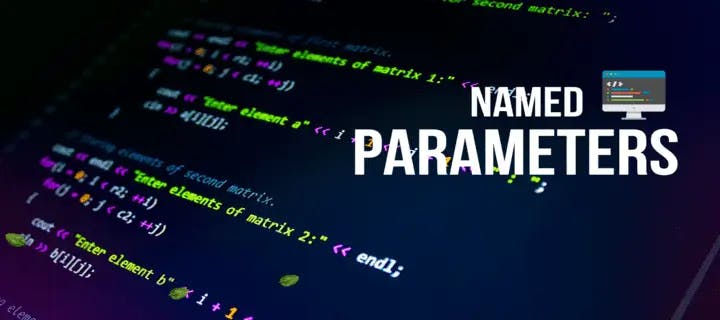
Named parameters: a modern and elegant approach
Introduction
When we are developing complex applications, a typical problem we may face is that we call a function or method that has many parameters, and we need to remember what the correct order is:
updateSomeState(oldState, newState, true, 0); // is this even right 🤔 ?
At this point you may think that we aren't separating the logic properly, and you may be right, but it's for example's sake.
A good approach to solving this problem is parameters destructuring (or named parameters). But first, let's review the destructuring syntax.
Basic destructuring
const IKodi = {
author: "Genaro Bonavita",
topics: ["programming", "JavaScript", "web development"]
};
const { author, topics } = IKodi; // creates two constants called 'author' and 'topics'
console.log(author); // "Genaro Bonavita"
console.log(topics[2]); // "web development"
Default values
If the properties we are looking for are not defined, the variables (or constants) are undefined
, but we can avoid this by setting a default value:
const { author = "Anonymous", topics = [] } = IKodi;
console.log(author); // if not defined, it will be assigned "Anonymous"
console.log(topics[2]); // if not defined, it will be assigned []
The default assignment only applies if the property is undefined
, meaning
that if it's null
(or any other falsy value) the created variable will be
assigned that no-undefined value.
Renaming destructured properties
Usually (for example when handling APIs responses), a property has the same name as one of our variables, or it's not descriptive enough (e.g. "data" or "response") and we want to rename it. We can rename the destructured property like so:
const { author: postAuthor, topics: postTopics } = IKodi;
console.log(postAuthor); // all OK 👍
console.log(postTopics[2]); // all OK 👍
console.log(author); // ReferenceError ⚠️ because doesn't exists
console.log(topics[2]); // ReferenceError ⚠️ because doesn't exists
Combined syntax
An example combining destructuring, default values and properties renaming:
const { author: postAuthor = "Anonymus", topics: postTopics = [] } = IKodi;
console.log(postAuthor); // "Genaro Bonavita", but "Anonymus" if not defined
console.log(postTopics[2]); // "web development", but [] if not defined
First we put the name of the original property, then the new name, and finally the default value.
Named Parameters
All these destructuring concepts apply to function parameters as well.
If you are using the modern parameter syntax explained above, you won't be able to use the use strict mode inside the function body.
// with normal parameters
function removeRepeatedItems(items) {
return Array.from(new Set(items));
}
// with named parameters
function removeRepeatedItems({ items = [] }) {
return Array.from(new Set(items));
}
The second removeRepeatedItems
function takes an object as argument and destructures the items property.
So, going back with the first example in this article, we can do the following:
updateSomeState({ oldState, newState, priority: true, destroyedNodes: 0 }); // this is more descriptive now
Wrapping Up
In this article, we learned the destructuring syntax, and combined it with named parameters, in order to have a better control over our code.
As you can see, it can be a useful approach when working on a large or complex codebase, because we're taking advantage of a native feature to reduce complexity, while improving the readability and maintainability of our code.
However, it is in your hands to decide if using it can help more than hinder. In the end, you have one more tool at your disposal.